PIN Capture
The SDK supports to capture PIN separated. This is mainly for SCA PIN capture.
PinPad Modes
We support three different PinPad modes [Shuffle, Moving and Fixed] with default UI as shown in the figure
enum class PinEntryMode {
SHUFFLE,
MOVING,
FIXED
}
Shuffle
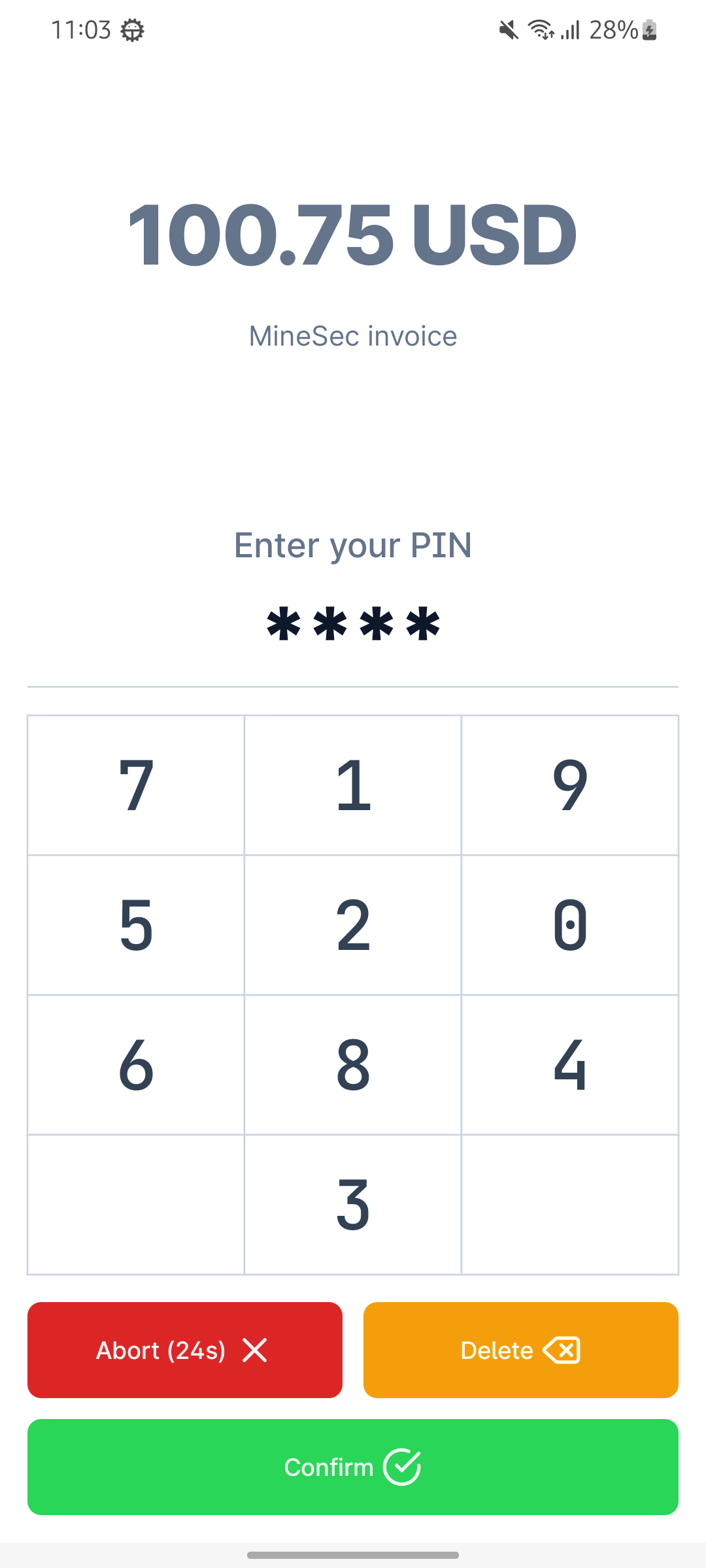
Moving
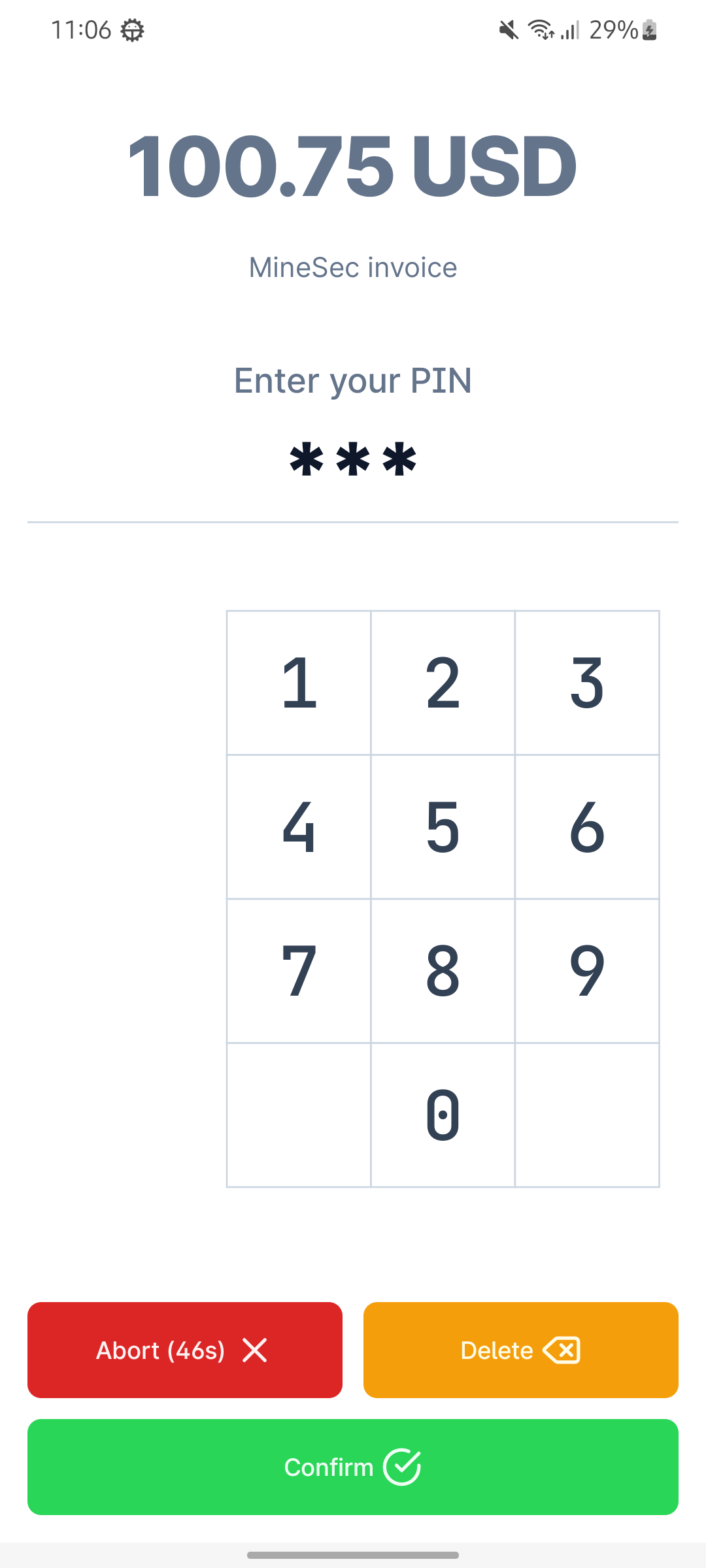
Fixed
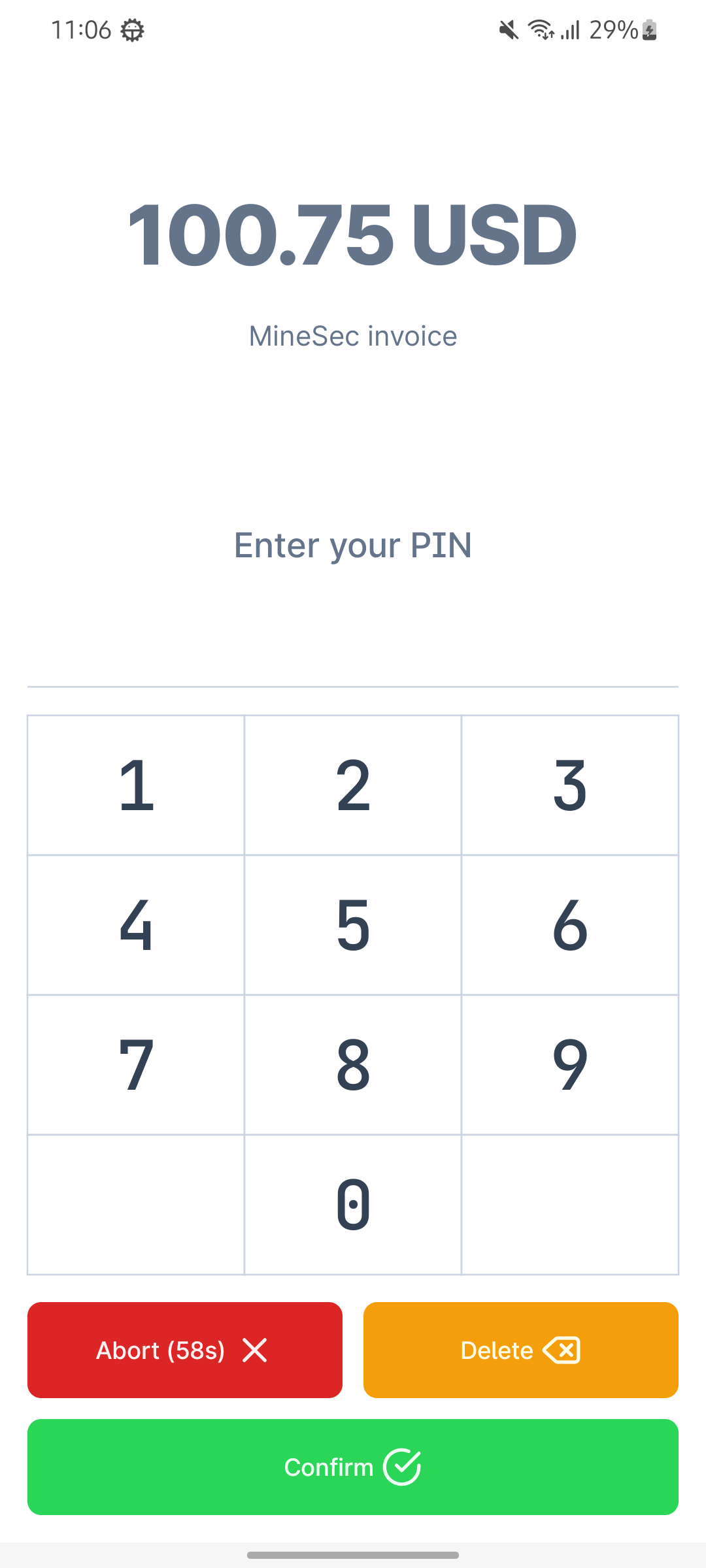
PIN Entry Details
The PIN entry screen require Amount to be passed alongside with other optional fields with default values shown below
data class PinEntryDetails(
val amount: String,
val description: String = "",
val pinDescription: String = "Enter your PIN",
val supportPINBypass: Boolean = true
)
SDK Customizations
You can customize all the fields shown in the following figure by having your own PinPadStyle instance, if you didn't use custom styling then default PinPad style screen will be rendered
data class PinPadStyle(
val backgroundColor: Int? = null,
val amountStyle: TextStyleConfig = TextStyleConfig(),
val descriptionStyle: TextStyleConfig = TextStyleConfig(),
val pinMessageStyle: TextStyleConfig = TextStyleConfig(),
val digitStyle: TextStyleConfig = TextStyleConfig(),
val buttonsColor: ButtonsColor = ButtonsColor()
)
data class TextStyleConfig(
val color: Int? = null,
val fontSize: Float? = null,
val fontFileUri: Uri? = null
)
data class ButtonsColor(
val abortBtn: Int? = null,
val clearBtn: Int? = null,
val confirmBtn: Int? = null,
)
Input parameters
- JSON encoded request with PAN and it should be a dummy PAN for capturing PIN BLOCK. when it's a empty string. MPoC SDK will look into memory cache to see if there is an encrypted PAN which is captured in the recent transaction. ( Note: this memory cached encrypted PAN is only valid for a limited time (1-2mins) )
val requestSCA = json.encodeToString(PinEntryDto("1122334455667788"))
- PinPadConfig instance to pass the required configrations for PIN screen
data class PinPadConfig(
val pinPadStyle: PinPadStyle = PinPadStyle(),
val pinEntryMode: PinEntryMode = PinEntryMode.SHUFFLE,
var pinEntryDetails: PinEntryDetails = PinEntryDetails("100.00 USD")
)
Launch PinPad Activity
- Add your json encoded request to a bundle.
- Add intent extras for the bundle and config instances.
Note: Below call mode is available starting from v2.01.001.011.029.
// we use intent name to replace "SecurePinPadActivity" class as this is removed from SDK list
val intent2=Intent("com.theminesec.minehades.PIN_ACTIVITY").apply {
// addFlags(Intent.FLAG_ACTIVITY_NEW_TASK) Note: YOU CAN NOT ADD THIS !!
putExtra(PinPadConstants.EXTRA_PIN_PAD_CONFIG, getPinPadConfig(context, selectedMode.value))
putExtra(PinPadConstants.BUNDLE_PIN_REQUEST, bundle)
}
Note: Below is only available on the version before v2.01.001.010.029
val bundle = Bundle()
bundle.apply {
putString(PinPadConstants.EXTRA_PIN_SCA_CAPTURE, requestSCA)
}
val intent = Intent(context, SecurePinPadActivity::class.java).apply {
putExtra(PinPadConstants.EXTRA_PIN_PAD_CONFIG, getPinPadConfig()
putExtra(PinPadConstants.BUNDLE_PIN_REQUEST, bundle)
}
- Launch the activity and observe its result
val launcher = rememberLauncherForActivityResult(
contract = ActivityResultContracts.StartActivityForResult()
) { result ->
if (result.resultCode == Activity.RESULT_OK) {
val response = result.data?.getStringExtra(PinPadConstants.EXTRA_PIN_CAPTURE_RESPONSE)
response?.let {
val pinResponse = json.decodeFromString<PinEntryResponse>(it)
}
} else if (result.resultCode == Activity.RESULT_CANCELED){
val errorCode = result.data?.getIntExtra(PinPadConstants.EXTRA_PIN_CAPTURE_RESPONSE_CODE, 0)
val errorMsg = result.data?.getStringExtra(PinPadConstants.EXTRA_PIN_CAPTURE_RESPONSE_MSG)
}
}
launcher.launch(intent)
Output parameters
data class PinEntryResponse(
val pinBlock: String,
val keyId: String,
val panLast4:String
)